toblick
/
cs101
/
basic computer concepts
class: center, middle # Basic computer concepts --- # Agenda 1. [Computers](#computers) - [Hardware](#hardware) - [Operating Systems](#operating_systems) - [Applications](#applications) 1. [Desktop Analogy](#desktop_analogy) - Files and good practices 1. [Networks](#networks) 1. [Numbers](#numbers) 1. [Conclusions](#conclusion) --- ## Computers Several levels: - Computer hardware - Operating system - Applications --- name: hardware ## Computer Hardware CPU Storage - Memory - Drives Input - Mouse - Keyboard - Camera - Microphone Output - Video - Speakers/Headphones I/O - Network --- ## CPU - Controls memory - Can read and execute commands - Modern processors schedule commands between multiple cores
--- ## Memory Data is stored in a complex sequence of switches called _bits_ -- Computers work 8 bits at a time called a _byte_ - In many languages a "character" is 1 byte -- It's the easiest place to store data in programs - Variables and "state" are typically stored in memory - CPUs have faster memory in "cache" (10x) - Drives can store much more, but are much slower (~1000x) Cache < Memory < Disk (numbers according to [Chandler Carruth in 2014](https://www.youtube.com/watch?v=fHNmRkzxHWs&t=2201)) --- ## System-on-Chip Some computers combine parts together
ars technica
--- ## Larger architectures
NYU High Performance Computing
--- name: operating_systems ## Operating systems Sits between hardware and users - Handles data input / output - Schedules which software has access to different resources and when --- ## Operating systems 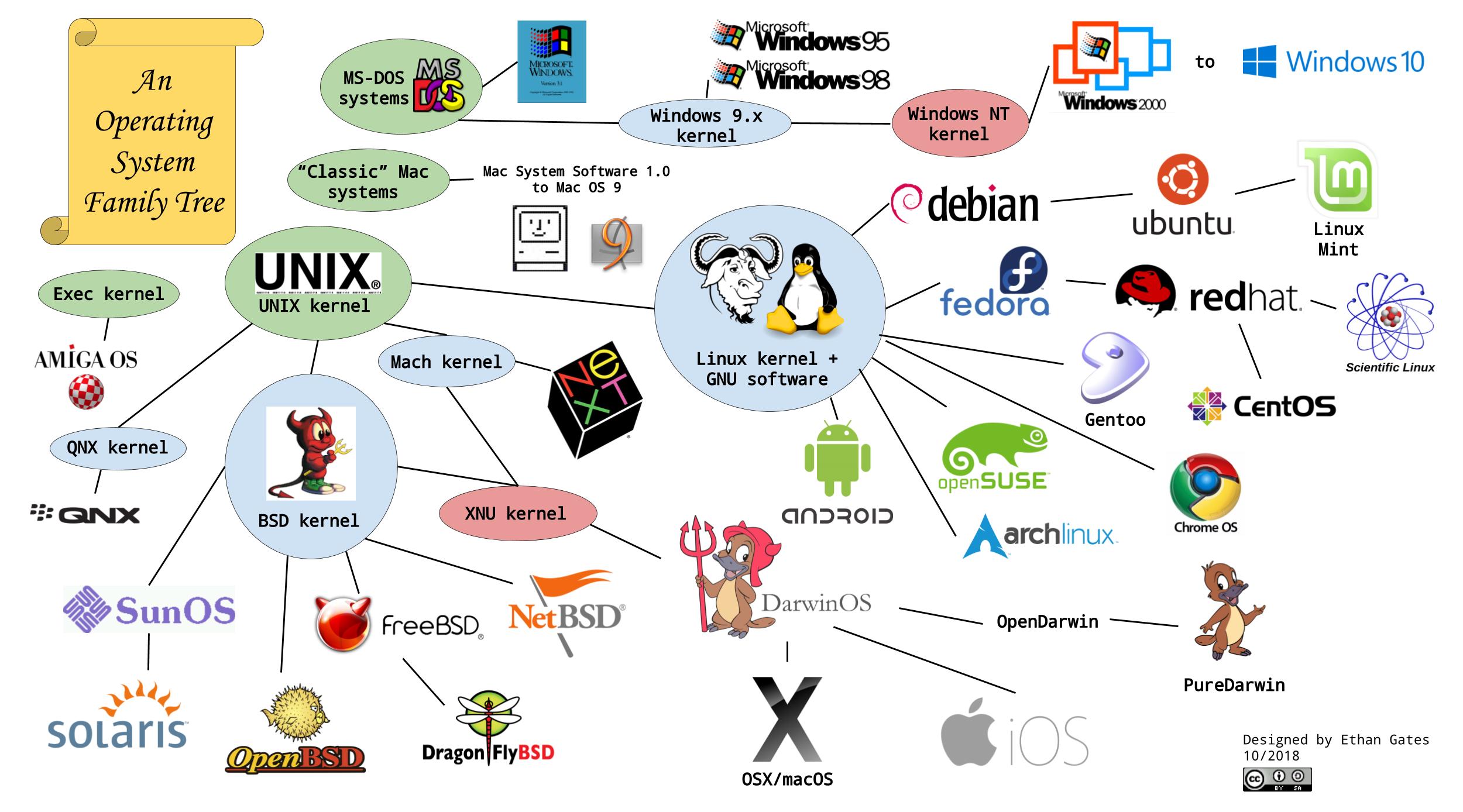 --- name: desktop_analogy ## Desktop Metaphor - How do computers store data? - All 0's and 1's, but we organize them using software so other software can derive meaning - We already had systems for organizing data at our desks, so we modeled off of them
.right[images are links to sources] --- name: files_and_folders ## Files and folders - Paper analogy - Files:
- Folders / Directories:
.right[Icons from [KDE Breeze icon set](https://github.com/KDE/breeze-icons) and cabinet from [Gnome Nautilus](https://wiki.gnome.org/action/show/Apps/Files?action=show&redirect=Apps%2FNautilus)] --- ## Types of files We might think of there as being many types of files, associated with differenet applications
Underneath, there are two fundamental kinds of files: - Text files - Binary files. -- Text files still use binary. --- ## Text files Lines of human-readable characters, potentially separated into lines. No colors, no formatting (italics/bold/etc), no images/media. Writing software (source code) almost always involve manipulating text files.
--- ## Creating and editing text files Multi-platform - Visual Studio Code - [Vi](https://ex-vi.sourceforge.net/) ( [VIM](www.vim.org) , [NeoVim](neovim.io)) - [Emacs](gnu.org/software/emacs) - [Sublime Text](sublimetext.com) - [QtCreator](www.qt.io/product/development-tools) Windows - [Visual Studio](https://visualstudio.microsoft.com/) - [Notepad++](https://notepad-plus-plus.org/) OSX - [XCode](developer.apple.com/xcode) - [TextMate](macromates.com) More language-specific - [JetBrains](jetbrains.com) products (IntelliJ IDEA, PyCharm, CLion, etc...) - [Eclipse](eclipse.org) --- ## Encoding Text Files - Text is "just" binary data - How do computers know what it means? - Text files follow an encoding for what each character means - Commonly: - ASCII ('A' = 65 = 1000001, 'B' = 66 = 1000010) - UTF-8 (Unicode Transformation Format – 8-bit) (some programming languages allow you to use Unicode characters as variables) - Other encodings - [link](https://en.wikipedia.org/wiki/Character_encoding#Common_character_encodings) - Different computers define new lines differently - [link](https://en.wikipedia.org/wiki/Newline#Representation) --- ## Examples of Text Files Source Code ```java class HelloWorld { public static void main(String[] args) { System.out.println("Hello World!"); } } ``` json ```json { "class": "CSCI-UA-0101-003", "professor": "Tobias Blickhan", "building": "WWH", "room": 402 } ``` --- ## Binary files - There are many other ways to store data - Numbers (from wiki on float32)
- Colors (from wiki on bitmap encoding)
- Specialized software might choose their own custom data to a file. - Saves space - Faster/easier for computers to read - Makes it difficult to open files without the right software - Examples: - Multimedia (.png/.jpg/.tif/.mp4) (not .svg!) - Programs (.exe) - Microsoft Office files (.docx/.xlsx/.pptx) --- ## Surprising Example of a Text File ```xml
``` --- ## Surprising Example of a Text File
--- ## Binary file editors Images - Raster (Pixel-based) - Photoshop (necessary for .psd files) - Gimp - Paint - Vector (line/curve based) - Illustrator (necessary for .ai files) - Inkscape Microsoft Office files - Better buy Office - Reverse engineered in OpenOffice / Google Workspace / Apple Pages - Occasional inconsistencies / issues - Missing features PDF Files - Better use Adobe software - Reverse engineered in libpoppler (okular/evince) or OSX Preview - Occasional inconsistencies / issues - Missing features --- ## Good File Naming Conventions Try to use lowercase without spaces ```bash file.zip file.Zip file.ZIP FiLe.ZiP ``` Avoid spaces in file names. ```bash important_document.js less_important_document.txt # 2 files important document.js less important document.txt # 5 files? ``` -- Or follow whatever
conventions
your group, OS, or language uses. --- ## File extensions How does your computer know which software opens a file? ```bash doc.docx doc.cpp doc.ai doc.pdf ``` -- This is all a convention, it's up to software to detect inappropriate files. ```python for filename in ["doc.docx", "doc.cpp", "doc.ai", "doc.pdf"]: with open(filename, "r") as fd print(fd.readlines()) ``` -- Your computer manages a list of default extensions. --- ## File permissions Computers are all designed by multiple "users": - The computer itself - Administrators - Normal users -- Every file and folder controls who can read/write/execute a file - user: the user who 'owns' the file or folder... every file or folder is owned by one user - group - a group of users who assigned to the file or folder - others - everyone else not including the user or the group --- ## The Environment Software needs some context for how it should run - Arguments - The input data that the user provided - Which input files/locations to open - What data should be generated? - Where/how should that be data stored? -- - Working Directory - Software is run from a particular directory for user convenience - "Relative paths" -- - Environment variables - Other data that the user or operating system has set - Which type of output is available - What encoding data comes in - What other software to use --- ## Representing numbers You might be familiar with the binary number system:
--- ## Representing numbers If our memory can store 0s and 1s (think: on/off or up/down), then we can represent an integer in binary: $$719 = 1011001111 = 2^9 + 2^7 + 2^6 + 2^3 + 2^2 + 2^1 + 2^0$$ 10 bits. This number can already not be represented in 8 bits: 0, 1, ..., 255. If you want to represent negative numbers, one bit has to hold the sign, so you are left with -128,-127,...,126,127. --- ## Representing numbers Adding numbers in binary is simple: just carry the ones. $$ 719 = 1011001111 = 2^9 + 2^7 + 2^6 + 2^3 + 2^2 + 2^1 + 2^0 $$ $$ 720 = 1011010000 = 2^9 + 2^7 + 2^6 + 2^4 $$ If all bits are 1s, then you loop around to 000...001 or something of the sort, which is either a very small or a negative number! [Wikipedia](https://en.wikipedia.org/wiki/Integer_overflow) has some examples of integer overflow bugs - from aviation to game consoles. --- ## Representing numbers This system obviously has its flaws. We need 80 bits to represent the Avogadro constant 6.02214076e23 (one holds the sign). Let's go back to [Float32 (IEEE 754 standard)](https://en.wikipedia.org/wiki/Single-precision_floating-point_format):
$$(-1)^{\mathrm{sign}} \times 2^{\mathrm{exponent}} \times 1.{\mathrm{fraction}} $$ Not all bits are treated equally now: One holds the sign, 8 hold the exponent, and 23 hold the fraction, i.e. the digits after the 1. They can represent \\(\ \{-1,+1\},\{-126,...,127\}, \{1,1+2^{-23},...,2-2^{-23}\} \\). Exponents with all ones and all zeros are reserved (Inf, NaN, 0). The smallest representable number is now \\(\ 2^{−126} × 2^{−23} \approx 1.4 \times 10^{-45} \\). The smallest number larger than one is \\(\ 1 + 2^{−23} \approx 1 + 1.2 \times 10^{-7}\\). Most calculations are done in double or Float64 format. --- ## How does a computer actually compute? At the lowest level: how do we add two bits? Input: two bits. Output: $$ \{0,0\} \mapsto \{ 0, \text{carry } 0\}. $$ $$ \{0,1\} \mapsto \{ 1, \text{carry } 0\}. $$ $$ \{1,0\} \mapsto \{ 1, \text{carry } 0\}. $$ $$ \{1,1\} \mapsto \{ 0, \text{carry } 1\}. $$ Call the two inputs A, B and the output S, C. You can translate this to: S = "A or (exclusive!) B". C = "A and B". If you can build a circuit (or a mechanical system!) that implements "exclusive or" as well as "and", you can add two bits! You can then chain these elements together to add more bits (have to consider carried bits as input now!). --- ## Transistors and Gates [A NAND gate](../files/nand.jpg) (from Wikipedia). Outout is "on" if and only if A and B are not both "on". Basic logic gates: NOT, AND, OR, XOR, NAND, NOR, NXOR. "XOR" refers to exclusive or: A OR B, but not both. --- ## Transistors and Gates [A half adder made from NAND gates](../files/half_adder.jpg) and [one made with an XOR and AND gate](../files/half_adder_xor.png) (also from Wikipedia) $$ \{0,0\} \mapsto \{ 0, \text{carry } 0\}. $$ $$ \{0,1\} \mapsto \{ 1, \text{carry } 0\}. $$ $$ \{1,0\} \mapsto \{ 1, \text{carry } 0\}. $$ $$ \{1,1\} \mapsto \{ 0, \text{carry } 1\}. $$ S = "A or (exclusive!) B". C = "A and B". --- ## Conclusion An idea for how components of a computer operate. A little excursion into number representation. A little excursion into how to build a bit adder.